Want to write clean and secure code? This guide to the top enterprise source code quality tools in 2023 will help you get started.
When software projects are driven by tight deadlines and urgent priorities, it’s tempting to prioritize speed over quality. However, hastily written, poorly structured code can quickly become a burden that hampers productivity and introduces more problems in the future.
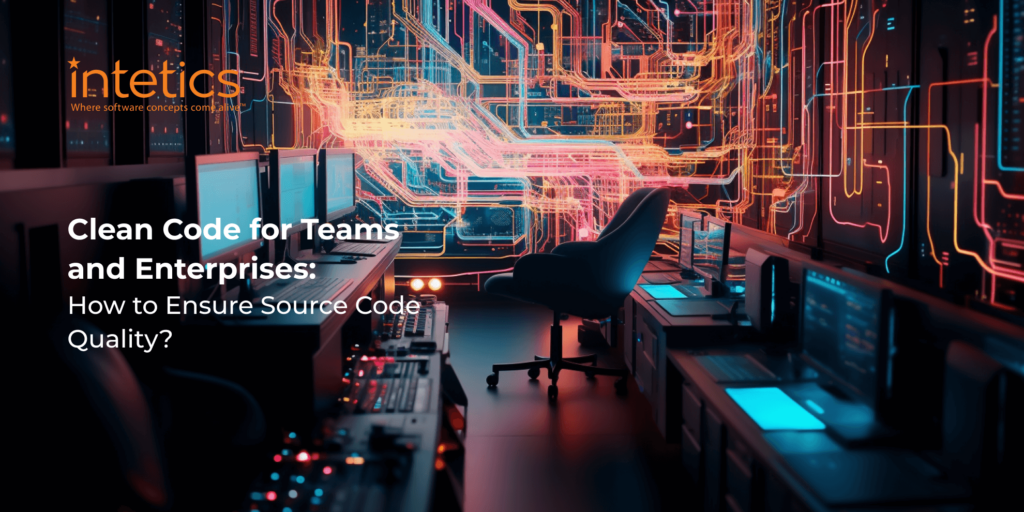
In fact, some estimates indicate that 20-40% of a developer’s time is wasted due to technical debt and bad code. So, the consequences of cutting corners in code will inevitably come back to bite you.
Related material: Technical Debt: No Biggie or a Threat to Your Business?
Busy development teams and enterprises are striving to establish a balance between delivering results quickly and maintaining high standards of code quality. Fortunately, there are ways to find harmony. Discover practical techniques for incorporating clean coding practices into day-to-day software development processes and explore the top source code quality tools.
What Is Source Code Quality?
Source code quality is a measure of how well the code is crafted and meets certain criteria. It’s a multidimensional concept that takes into account various factors, characteristics, and practices related to the development, maintenance, and usability of the codebase.
Here are some key aspects to consider when evaluating code quality:
- Being bug-free and error-free: delivers the intended results consistently.
- Performance and efficiency employ efficient algorithms, data structures, and coding techniques.
- Security: ensures the confidentiality, integrity, and availability of sensitive data; addresses identified security vulnerabilities.
- Reusability: allows developers to extract and reuse specific components or functions in different parts of the codebase or even in other projects.
- Readability: employs consistent naming conventions, appropriate comments, proper indentation, and manageable lines of code.
- Testability: exhibits such characteristics as modularity, loose coupling, and proper separation of concerns.
- Meeting client requirements: it fulfills the intended purpose, meets user expectations, and delivers the desired functionality.
- Documentation: includes comments within the code, as well as external documentation that describes the code’s functionality, APIs, and interfaces.
It is risky and not recommended to wait until just before the live release to assess the quality of your product’s source code. Instead, you should proactively address code quality throughout the development lifecycle.
How to Ensure Source Code Quality
Want to make the code easier for your team and other stakeholders to work with? Here are some practices you may follow:
Code Reviews
After a software developer completes their coding tasks, a code review provides an opportunity to have a second opinion on the solution and implementation. During a code review, the reviewer thoroughly examines the code to find bugs, logic problems, uncovered edge cases, or any other issues that may have been missed. The reviewer’s role is not just limited to finding defects, though; they also act as a gatekeeper ensuring the code aligns with best practices and architectural guidelines.
The choice of a reviewer is crucial. Ideally, they should be a domain expert who possesses a deep understanding of the specific problem domain being addressed by the code. If the code spans multiple domains, it’s better to involve multiple experts.
Examples of Code Review Outcomes
These are just a few examples of the types of feedback and suggestions that can arise during a code review. The goal is to provide constructive criticism and collaborate with the developer to enhance the codebase and overall software quality.
- Code duplication: “There is duplicate code in these two functions. Let’s refactor it into a helper function to improve maintainability.”
- Error handling: “What happens if the database connection fails? Please add proper error handling and fallback mechanisms.”
- Security vulnerabilities: “Make sure to sanitize user inputs to prevent potential SQL injection attacks.”
- Test coverage: “Consider adding additional test cases to cover different scenarios, especially edge cases and corner cases.”
- Modularity and reusability: “This code could be refactored into smaller, reusable functions to improve code modularity and maintainability.”
Consistent Coding Standards
At an individual level, consistency means following a set of coding conventions consistently within your enterprise’ code. There should be a specific code formatting style, naming conventions, and other coding practices that you find most comfortable and effective.
In a collaborative development environment, you also need collective consistency. By respecting and following the existing code style prevalent in the files you touch, you make it easier for other developers to read and work with the code.
And you can’t ignore industry-wide consistency if you recognize that adopting shared coding conventions and practices benefits the software development community as a whole. So, the code needs to be easily understood and maintained by developers from different backgrounds and organizations.
Common coding conventions may cover the following areas:
- Comment conventions
- Indent style conventions
- Line length conventions
- Naming conventions
- Programming practices
- Programming principles
- Programming style conventions
Some of the industry-known coding standards include the CERT C Coding Standard, MISRA C, High Integrity C++, etc.
Automated Testing
Automated testing provides a systematic and efficient way to verify the correctness, functionality, and performance of software applications. It covers such aspects of code quality as performance testing, code style checks, error-prone code detection, compatibility testing, and identification of unused code.
Moving beyond these capabilities, the incorporation of AI testing significantly refines this process. The application of AI introduces a deeper level of analysis, capable of uncovering intricate patterns and pinpointing errors that might elude traditional testing methods. Functionize’s insights on AI testing show that it not only enhances the precision of tests but also streamlines the identification of potential issues, ensuring software resilience and user satisfaction. As AI-driven approaches evolve, they promise to revolutionize the efficiency and effectiveness of automated testing, setting a new standard for software quality assurance.
Let’s consider a web application that allows users to register and log in. Without automated testing, developers would manually test the registration and login features after making changes to the codebase. However, with the introduction of automated tests, the process becomes much more robust:
- Test Coverage: Automated tests can provide extensive test coverage by systematically checking various scenarios and edge cases. For example, a suite of automated tests can be created to verify that a user can successfully register, log in, handle different password requirements, and handle error conditions such as invalid email addresses or duplicate usernames.
- Regression Testing: Automated tests can be rerun automatically every time there are code changes or new feature additions. This helps catch any regressions or unintended side effects caused by modifications to the codebase. For instance, if a developer adds a new feature that inadvertently breaks the login functionality, the automated tests will detect the issue, alert the team, and prevent the faulty code from being deployed to production.
Of course, the biggest benefit of automated testing is its ability to save time and effort on continuous inspections. But these tools aren’t infallible. False positives or false negatives can occur, so developers need to carefully analyze the results and perform additional manual reviews when necessary.
Test Coverage
Test coverage defines what percentage of application code is tested and whether the test cases cover all the code.
For example, if you have 10,000 lines of code, your test cases should be able to test the entire codebase. If only 5,000 lines of code are tested out of 10,000, the coverage is 50%.
Higher test coverage means more of the code is tested, reducing the risk of undiscovered issues or bugs. Without sufficient test coverage, untested portions of the code may contain hidden bugs. By aiming for higher test coverage, developers can have greater confidence in the quality of their codebase and detect potential issues early on, leading to more reliable software.
At the same time, test coverage addresses one of the common challenges in testing, which is dealing with unnecessary or redundant test cases. By analyzing test coverage, developers identify and eliminate these redundant cases, allowing them to focus their testing efforts on areas that require attention.
Moreover, test coverage helps align the testing efforts with the requirements specified in documents like the Functional Requirements Specification (FRS), Software Requirements Specification (SRS), and User Requirement Specification (URS).
Refactoring
Refactoring transforms messy, incorrect, or repetitive code into clean and well-structured code with reduced complexity. It tackles the challenges that arise when multiple developers work on code, which may danger consistency and standardization across the project.
When developers refactor their code, it becomes easier to read, understand, and maintain, which improves its overall quality. And the removal of unnecessary elements, such as code duplications, results in optimized memory usage and improved performance. Another benefit is that refactored code provides a solid foundation for future development and expansion, as it’s more flexible and adaptable to accommodate new features.
Code Refactoring Examples
There are dozens code refactoring examples, but let’s focus on several of them:
Remove Dead Code
When software requirements change or corrections need to be made, it’s common for old code to be left uncleaned due to time constraints. This can include dead code.
The quickest way to find dead code is to use a good IDE.
- Delete unused code and unnecessary files.
- In the case of an unnecessary class, Inline Class or Collapse Hierarchy can be applied if a subclass or superclass is used.
- To remove unneeded parameters, use Remove Parameter.
Extract Class
To move functionality from a large, monolithic class to a smaller, more focused class, the Extract Class refactoring technique is used. It means creating a new class and placing the fields and methods responsible for the relevant functionality in it.
Compose Method
Excessively long code is hard to understand and hard to change. The Compose method refers to a range of actions that can be used to streamline methods and remove code duplication. These include Inline Method, Inline Temp, Replace Temp with Query, split temporary variables, and remove assignments to parameters.
Modularity and Reusability
Modularity involves breaking code into smaller, self-contained modules to promote organization and maintainability. This separation can be achieved through various patterns, from the obvious ones, like separating the front-end, back-end, and database layers, to more niche patterns, like vertical slices or the mediator pattern.
As for reusability, it means creating components that can be reused across projects to save time and enhance consistency. It prioritizes:
- Abstraction (to hide implementation details and provide a simplified interface).
- Generality (to ensure modules are applicable to various situations through parameters and configurations).
- Extensibility (to allow easy modification without breaking functionality).
Also, standard libraries, frameworks, and patterns that offer reusable functionality are great ways to harness collective knowledge.
Error Handling and Logging
There should be robust mechanisms to gracefully handle exceptions and errors—catching and handling exceptions at appropriate levels, providing meaningful error messages, and taking appropriate actions to recover from or mitigate the error. This ensures that errors are handled in a controlled manner, which ultimately helps with preventing application crashes and unexpected behavior.
Additionally, logging plays a crucial role. Logging provides a record of events (the exact date and time, the location where the error occurred, a severity level, descriptive text that explains the event, plus additional contextual information), which allows developers to trace the sequence of actions leading up to the error and understand the state of the system at the time. This information is invaluable in diagnosing and resolving issues.
Documentation
Code documentation examples include comments within the code, external documentation such as user manuals, technical specifications, design documents, and internal documents like coding guidelines, standards, and conventions.
While some may argue that good code is self-explanatory, accurate documentation is an essential component of a high-quality codebase. Firstly, it allows developers to quickly understand what the code does and how to work with it. Clear and concise documentation significantly reduces the time spent on deciphering code, especially for new team members or developers working on legacy projects.
Secondly, writing documentation has a positive impact on the codebase itself. The process of documenting code forces developers to articulate their thinking and logic, which may point out areas of improvement. Documentation also helps uncover overly complicated parts of the code, thus encouraging refactoring and better architectural decisions.
Version Control and Branching
Version control systems like Git provide a centralized repository for tracking and managing code changes, which developers need to maintain a complete record of every modification with details, such as authorship and timestamps. This transparency aids in identifying and resolving issues introduced during development.
In a similar vein, feature branching enables isolated development of new features, reducing the risk of introducing bugs to the main codebase. GitFlow, for example, provides a structured workflow for managing software releases and bug fixes, which ensures proper review and testing before merging changes.
Continuous Integration and Deployment (CI/CD)
This development philosophy emphasizes frequent code integration and automated processes. By practicing CI/CD, developers regularly commit their code to the version control repository, often on a daily basis. It allows for early detection of defects and other quality issues, as smaller differentials are easier to analyze and troubleshoot compared to larger code changes developed over a longer period.
On a more technical level, CI/CD involves setting up automated build and testing pipelines that are triggered whenever code changes are committed. These pipelines automatically compile the code, run tests, and perform other quality checks. The result is faster feedback and fewer bugs.
Performance Optimization
Imagine a web application’s source code that displays a list of products retrieved from a database. Initially, the code retrieves all the products from the database and processes them directly in the presentation layer before rendering them on the webpage. However, with the growing number of products, this approach leads to slow page loading times and decreased user experience.
Good source code is not only about writing functional and bug-free code but also about ensuring that the code performs well in terms of speed, resource utilization, and scalability.
When code is optimized for performance, it undergoes a thorough examination to identify areas that may cause performance bottlenecks. By analyzing and improving these areas, for example, through techniques like reducing redundant operations, improving algorithmic efficiency, and optimizing resource utilization, developers make the code better. After all, a developer’s expertise should be not only crafting code that functions correctly but also performs optimally.
Peer Collaboration and Knowledge Sharing
Peer collaboration and knowledge sharing help disseminate valuable insights and expertise throughout the team. Developers can share their experiences, discuss innovative approaches, and suggest improvements to existing code. This goes back to having multiple sets of eyes on the code, plus it creates opportunities to learn from different coding styles and techniques.
But it also extends beyond code reviews. Pair programming is another beneficial practice where two developers collaborate on the same code, actively discussing and solving problems in real-time. And when more people enter the picture, like in open-source projects, it can even create a sense of collective responsibility for code quality. This makes the team more invested in maintaining high standards (assuming everyone shares the same commitment).
Flexibility & Governance: Top Enterprise Code Quality Tools in 2023
In the quest for impeccable source code, developers must recognize the paramount importance of source code analysis tools. Fortunately, there is a plethora of powerful tools to help them in this endeavor. We’ll roughly divide them into five categories, with several program suggestions in each.
Static Code Analysis Tools
A static code analyzer examines the source code of a program without actually executing it. Such tools offer suggestions for code refactoring, identify potential security vulnerabilities, and help enforce coding best practices. The goal is to catch and address issues early in the development process, minimizing the chances of those issues causing problems during runtime.
Some popular tools for static application security testing are:
- SonarQube (29 programming languages)
- ESLint (for JavaScript)
- Checkstyle (for Java)
- PyLint (for Python)
- RuboCop (for Ruby)
- PHP_CodeSniffer (for PHP)
Code Review Tools
Code review tools facilitate collaborative code review among team members by providing a platform for developers to share their code with peers, review it for issues, offer suggestions, and ensure adherence to best practices. These tools usually include features like commenting, discussion threads, and version control integration to streamline the review process and promote smoother collaboration.
Examples of widely used ones:
- GitHub (supports code review through pull requests)
- GitLab (includes built-in code review features)
- Bitbucket (provides code review capabilities)
Code Coverage Tools
As already discussed briefly, code coverage tools measure the extent to which the source code is exercised by the test suite. They track which parts of the code are executed during testing and provide metrics to assess the coverage achieved. In other words, they help evaluate the thoroughness of the testing process by identifying areas of code that are not adequately tested.
If you’re considering using such a tool, here are some options:
- JaCoCo (for Java)
- Istanbul (for JavaScript)
- coverage.py (for Python)
- PHPUnit (for PHP)
Linters
Linters primarily focus on code formatting, style consistency, and catching simple errors that can be detected statically. They provide immediate feedback during development and help maintain clean and consistent code.
Some of the commonly used linters are:
- ESLint (for JavaScript)
- Pylint (for Python)
- RuboCop (for Ruby)
- PHP_CodeSniffer (for PHP)
Dependency Analysis Tools
These tools help focus on managing and analyzing software dependencies between various components, libraries, and frameworks within a project. They can identify outdated or vulnerable dependencies, detect compatibility issues, and provide insights into the impact of making changes. You don’t want to overlook this because compatibility issues between different dependencies can cause conflicts and result in runtime errors or unexpected behavior.
Here are a few examples of open-source tools for dependency analysis:
- OWASP Dependency-Check
- Snyk
- Black Duck
Note: the choice of tools depends on the programming language and the specific requirements of your project. Many enterprise software development teams find it beneficial to use a combination of tools as they can get a more comprehensive analysis of the source code quality.
Featured Material: Defining and Tracking the Code Quality
Final Words
Don’t let the disconnect between the business and technical sides ruin the potential of your project. At the end of the day, the same business personnel who are pressuring developers to produce more deliverables without considering code quality will eventually return to them with modification requests for the same codebase. Or worse, they expect these changes to be trivial because the development team has already implemented certain parts of the feature.
Remember that investing in source code quality is investing in the long-term success of your software. By maintaining high-quality code standards, you pave the way for increased productivity, reduced technical debt, and improved customer satisfaction.
Source code quality and open-source code use are just a couple of areas Software Product Excellence by Intetics helps to assess. You can gain access to a suite of features and capabilities that go beyond simply highlighting code issues. If you’re aiming to create a future-proof product, this can be a valuable addition to your software development process. Let’s talk and find out how.
FAQ
What Are the Tools to Check Source Code Quality?
Some of the most widely used tools in the realm of code quality analysis are SonarQube, Checkstyle, ESLint, GitLab, Bitbucket, Pylint, etc.
What Is Source Code Quality?
Source code quality is the measure of how well-written, structured, readable, maintainable, and efficient the source code is. It reflects the overall quality of the codebase and its adherence to industry best practices and coding standards.
How Do You Analyze Source Code?
Source code analysis involves analyzing the code structure, syntax, and semantics to identify potential issues, bugs, vulnerabilities, and code smells. The process usually combines manual review and automated analysis to save time but still to achieve comprehensive results.